opencv-mobile
Introduction
opencv-mobile is a lightweight version of the OpenCV library that minimizes the compilation of OpenCV by adjusting compilation parameters and removing certain portions of the OpenCV source code.
opencv-mobile provides commonly used functionalities of OpenCV, such as image processing, matrix operations, and more. It stays synchronized with the upstream version and has no third-party dependencies. In the majority of cases, it can seamlessly replace the official OpenCV with only 1/10th of the size, making it particularly suitable for mobile and embedded environments with specific size requirements.
Source code package size comparison:
OpenCV 4.9.0 | The Official OpenCV | opencv-mobile |
---|---|---|
source zip | 93 MB | 10.7 MB |
android | 242 MB | 18.1 MB |
ios | 202 MB | 10.0 MB |
ios+bitcode | missing :( | 34.5 MB |
Project link: https://github.com/nihui/opencv-mobile | Thanks to nihui
!
opencv-mobile already supports hardware-accelerated JPG decoding and VPSS (Video Processing Subsystem) hardware acceleration on Milk-V Duo/Duo256M/DuoS.
1. Quick start
We can directly download its release precompiled package to test basic functions or carry out application development.
A test example is provided in the project source code, demonstrates how to use opencv-mobile to load images, zoom, and save images.
Taking this test program as an example, we will introduce the compilation method of opencv-mobile in Linux environment and how to run it on Milk-V Duo.
Download pre-compiled package for Milk-V Duo
The release link of opencv-mobile: https://github.com/nihui/opencv-mobile/releases
Download the latest pre-compiled package of Milk-V Duo: opencv-mobile-4.9.0-milkv-duo.zip
Create a new test program directory
Create a new directory picture-resize
and enter it:
mkdir picture-resize
cd picture-resize
Extract the pre-compiled package downloaded to the current directory:
unzip ../opencv-mobile-4.9.0-milkv-duo.zip
Create code file
Create a new file named main.cpp
:
vi main.cpp
Add the following:
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
int main()
{
cv::Mat bgr = cv::imread("in.jpg", 1);
cv::resize(bgr, bgr, cv::Size(200, 200));
cv::imwrite("out.jpg", bgr);
return 0;
}
Its function is to scale an image named in.jpg
to a size of 200x200
and then output it as an out.jpg
file.
Create CMakeLists.txt
To compile using cmake, you need to create a CMakeLists.txt file:
vi CMakeLists.txt
The content is as follows:
project(opencv-mobile-test)
cmake_minimum_required(VERSION 3.5)
set(CMAKE_CXX_STANDARD 11)
set(CMAKE_C_COMPILER "${CMAKE_CURRENT_SOURCE_DIR}/host-tools/gcc/riscv64-linux-musl-x86_64/bin/riscv64-unknown-linux-musl-gcc")
set(CMAKE_CXX_COMPILER "${CMAKE_CURRENT_SOURCE_DIR}/host-tools/gcc/riscv64-linux-musl-x86_64/bin/riscv64-unknown-linux-musl-g++")
set(CMAKE_C_FLAGS "${CMAKE_C_FLAGS} -D_LARGEFILE_SOURCE -D_LARGEFILE64_SOURCE -D_FILE_OFFSET_BITS=64")
set(CMAKE_EXE_LINKER_FLAGS "${CMAKE_EXE_LINKER_FLAGS} -mcpu=c906fdv -march=rv64imafdcv0p7xthead -mcmodel=medany -mabi=lp64d")
set(OpenCV_DIR "${CMAKE_CURRENT_SOURCE_DIR}/opencv-mobile-4.9.0-milkv-duo/lib/cmake/opencv4")
find_package(OpenCV REQUIRED)
add_executable(opencv-mobile-test main.cpp)
target_link_libraries(opencv-mobile-test ${OpenCV_LIBS})
There are three variables that need to be noted and configured according to your own file path:
- OpenCV_DIR: The directory corresponding to the precompiled package previously extracted to the current directory. Pay attention to the version number in the path.
- CMAKE_C_COMPILER: The path to gcc in the cross-compilation tool chain.
- CMAKE_CXX_COMPILER: The path to g++ in the cross-compilation tool chain
Download link for cross-compilation toolchain: host-tools.tar.gz. You can download it through the wget command then unzip:
wget https://sophon-file.sophon.cn/sophon-prod-s3/drive/23/03/07/16/host-tools.tar.gz
tar -xf host-tools.tar.gz
If you have ever compiled duo-buildroot-sdk, the host-tools
directory under its root directory is the directory of the cross-compilation toolchain. There is no need to re-download it. You can directly modify OpenCV_DIR
and specify it to this directory. Or create a soft link pointing to the directory.
Compile
Compiling in cmake mode will create some intermediate directories and files, so we create a new build
directory and enter this directory to complete it:
mkdir build
cd build
cmake ..
make
The normal compilation output is as follows:
$ make
[ 50%] Building CXX object CMakeFiles/opencv-mobile-test.dir/main.cpp.o
[100%] Linking CXX executable opencv-mobile-test
[100%] Built target opencv-mobile-test
The opencv-mobile-test
generated in the current build
directory is the test program:
$ ls
CMakeCache.txt CMakeFiles cmake_install.cmake Makefile opencv-mobile-test
The directory structure at this time is as follows:
picture-resize/ # Test program root directory
├── build # Compile output directory
├── CMakeLists.txt # CMake configuration file
├── host-tools # Duo cross-compilation tool chain directory
├── main.cpp # Test program source code file
└── opencv-mobile-4.9.0-milkv-duo/ # opencv-mobile precompiled library directory
Transfer the generated opencv-mobile-test
program to Duo through the scp
command:
scp opencv-mobile-test [email protected]:/root/
Then transfer a jpg
picture with a size of 1024x1024
for testing to Duo:
scp in.jpg [email protected]:/root/
in.jpg
:
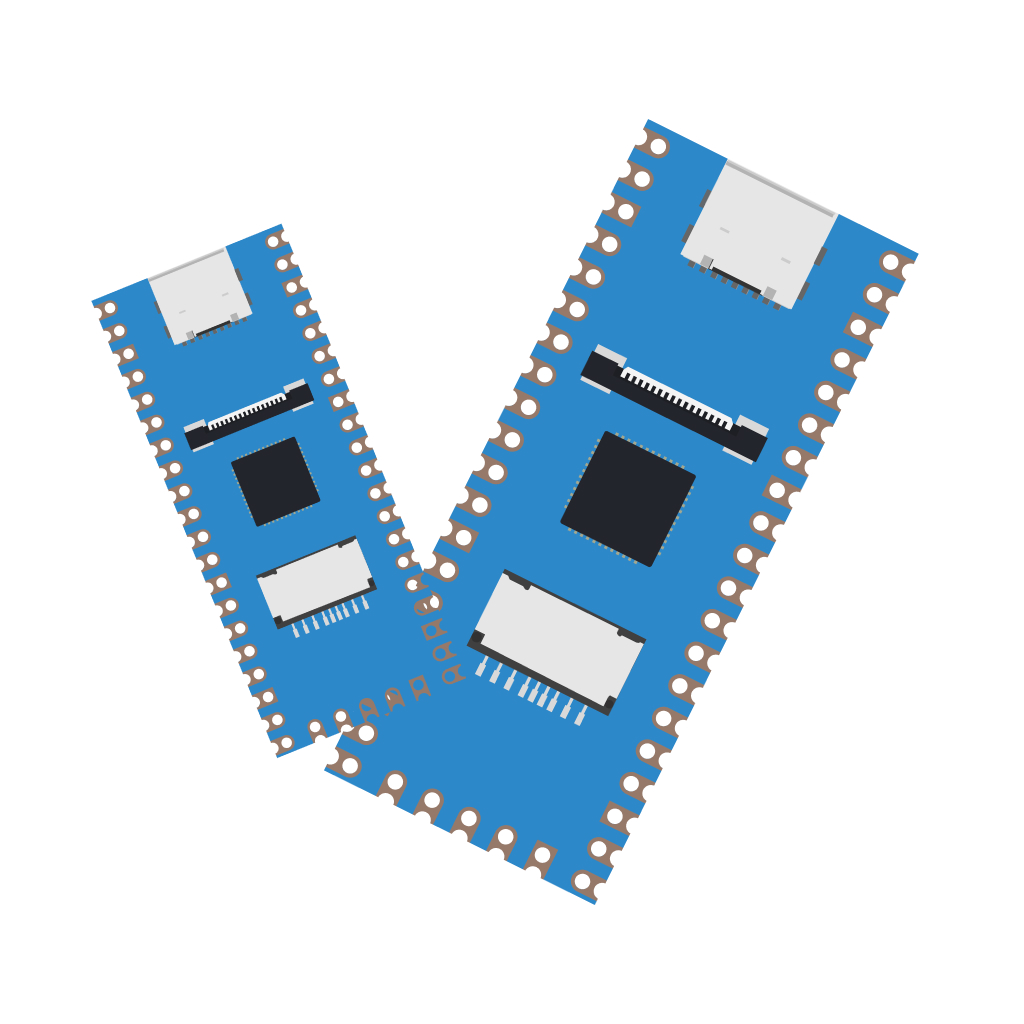
Run the test program in Duo
For Duo firmware, please temporarily use the version V1.0.8
Log in to the Duo terminal through the serial port or ssh and enter the /root/ directory:
cd /root/